
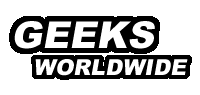
- Welcome to Geeksww.com
Bundle, validate, compress javascript files
I recently worked on a hybrid mobile app using PhoneGap. The plan now is to migrate the app to a mobile+desktop web app/site.
Due to a number of javascript files and libraries I had included in my project (and custom code written myself), I realized that I should do something to minimize the number of HTTP requests to server by bundling up source files somehow. I used simple OS shell commands to do so on a Mac OSX but instructions should work fine on Linux (with or without some adjustments).
One of the options was to use an automated solution like Gulp, Grunt etc but I wanted something really simple and quick. So, I came up with the strategy (explained below) that works for me.
How to validate and compress JS files:
Also, I'd like to mention here that I am going to be using Google's closure compiler to validate and minify/compress JS file. The benefit of using closure is that it finds obvious bugs in your code and in fact it did find some issues with my code that weren't compatible with IE8 and less. In short, its good to run your files through closure compiler.
How to make a list of JS files from multiple JS script tags:
I put all <script> tags in a text file and took out list of files (with relative paths) using text editor and put the list of filenames in a text file. Here is an example:
I initially had a list of script tags like below:
<script type="text/javascript" src="js/plugins.js"></script> <script type="text/javascript" src="js/load_templates.js"></script> <script type="text/javascript" src="js/app_events.js"></script> <script type="text/javascript" src="js/database_layer.js"></script> <script type="text/javascript" src="js/app.js"></script>
This list was converted into the following (by removing script tags):
js/plugins.js js/load_templates.js js/app_events.js js/database_layer.js js/app.js
I put the list above in a file named js_list.txt (so your webserver does not serve the .txt file, but I also recommend putting it outside of web server's document root).
I ran the following command to merge contents of all files listed in js_list.txt into a single .js file named tmp.txt. You can use a different file name (if you will).
cat js_list.txt | xargs cat > ../tmp.txt
How to remove whitespaces from each line:
If you want to make sure each line gets trimmed (to remove whitespaces from both ends of each javascript file name), use the modified command below.
cat js_list.txt | sed 's/^ *//g' | sed 's/ *$//g' | xargs cat > ../tmp.txt
How to add comments to ignore some files from inclusion in final merged file:
You can also add comments on each line by putting # at the beginning of lines. Javascript files on commented lines won't be included in the final bundled JS file.
Here is how I did that:
cat js_list.txt | sed 's/^ *//g' | sed 's/ *$//g' | egrep -v ^# | xargs cat > ../tmp.txt
Explanation on command:
Basically, the command above:
- takes each line of text file js_list.txt (containing the list of javascript file names)
- trims that line (removes whitespaces from both ends of the line)
- ignores it if it's commented out (ie. first non-whitespace character on the line is #)
- then fetches content of the JS file and
- finally appends it to an output text file named tmp.txt (can be changed, if needed)
How to compile, validate, and compress the final JS file using closure:
Once you have the final output file, use Google closure compiler (downloaded from https://developers.google.com/closure/compiler/) to validate and compress/minify it.
java -jar ~/compiler-latest/compiler.jar --js ../tmp.txt --js_output_file compiled.js
Make sure you adjust file paths in command above according to your setup.
If there are no errors, we should have the final compressed and bundled JS file compiled.js (name can be changed in the command above).
How to use separate JS files (script tags) during development:
In case, you're wondering what to do during development when you want to know which of the source files had the issue and write modular code. I used the following command to generate a list of script tags to be included into my development code.
cat ../js_list.txt | sed 's/^ *//g' | sed 's/ *$//g' | egrep -v ^# | xargs -I {} echo '<script type="text/javascript" src="{}"></script> '
You could also modify the command above to replace .js with .min.js to include minified versions of each files.
cat ../js_list.txt | sed 's/^ *//g' | sed 's/ *$//g' | egrep -v ^# | sed 's/.js/.min.js/g' | xargs -I {} echo '<script type="text/javascript" src="{}"></script> '
This works similar to the normal process of including each JS file in a separate script tag.
Did this tutorial help a little? How about buy me a cup of coffee?
Please feel free to use the comments form below if you have any questions or need more explanation on anything. I do not guarantee a response.
IMPORTANT: You must thoroughy test any instructions on a production-like test environment first before trying anything on production systems. And, make sure it is tested for security, privacy, and safety. See our terms here.
tags cloud
popular searches
free download for mysql database server 5.1.5, bison, gearman, source code, php, laptop, mysql, install cairo, java, linux, install mysql, mysql initialization, mysql mysql, tools, ubuntu
Similar Tutorials:
- Giving Apache Web Server A Different Name by changing Source Code Before Installation (very simple instructions)
- How to setup node.js/express.js, compass, bootstrap project
- How to write a function that generates random string in Javascript?
- Starting with Git - Setting up remote environment for Git
- PHP Useful functions (Part 1) - The inRange function
Tutorials in 'Web Development > Javascript' (more):
- How to write a function that generates random string in Javascript?
- Which frontend framework should i learn?
- How to execute a function at regular intervals using Javascript
- Go to Top of the Page Using Javascript
- Sleep/Pause for specified number of seconds
Comments (write a comment):
0 comments so far. Be the first one to leave a comment on this article.