
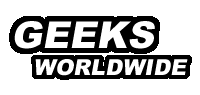
- Welcome to Geeksww.com
How to create and maintain a fixed length queue in dart?
In Dart, you can create and maintain a fixed length queue using the ListQueue class from the dart:collection
library. The ListQueue
class implements a queue using a list, and you can set the maximum capacity of the queue using its constructor.
Here's an example of how to create and maintain a fixed length queue in Dart:
import 'dart:collection'; void main() { // Create a fixed length queue with a maximum capacity of 5. var queue = ListQueue(5); // Add some elements to the queue. queue.add(1); queue.add(2); queue.add(3); // Print the contents of the queue. print(queue); // Output: (1, 2, 3) // Add more elements to the queue to exceed its maximum capacity. queue.add(4); queue.add(5); queue.add(6); // Print the contents of the queue again. print(queue); // Output: (2, 3, 4, 5, 6) // Remove an element from the queue. queue.removeFirst(); // Add another element to the queue. queue.add(7); // Print the contents of the queue again. print(queue); // Output: (3, 4, 5, 6, 7) }
In this example, we create a ListQueue with a maximum capacity of 5 by passing 5 as an argument to its constructor. We then add some elements to the queue and print its contents.
Next, we add more elements to the queue to exceed its maximum capacity. The oldest element is automatically removed from the queue to maintain its fixed length.
Finally, we remove an element from the queue, add another element, and print its contents again. The queue continues to maintain its fixed length of 5 elements.
Did this tutorial help a little? How about buy me a cup of coffee?
Please feel free to use the comments form below if you have any questions or need more explanation on anything. I do not guarantee a response.
IMPORTANT: You must thoroughy test any instructions on a production-like test environment first before trying anything on production systems. And, make sure it is tested for security, privacy, and safety. See our terms here.
tags cloud
popular searches
free download for mysql database server 5.1.5, bison, gearman, source code, php, install cairo, java, mysql, install mysql, laptop, linux, mysql mysql, mysql initialization, tools, ubuntu
Similar Tutorials:
- Dart - x positional argument(s) expected, but 0 found.
- ?? - the null-coalescing operator in Dart
- Options to store data locally in a flutter app
- How to create a constructor that clones another object in Dart
- Pass an object through navigator.pushnamed using provider
Tutorials in 'Web Development > Dart' (more):
- Options to store data locally in a flutter app
- Dart - x positional argument(s) expected, but 0 found.
- ?? - the null-coalescing operator in Dart
- Pass an object through navigator.pushnamed using provider
- How to create a constructor that clones another object in Dart
Comments (write a comment):
0 comments so far. Be the first one to leave a comment on this article.