
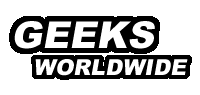
- Welcome to Geeksww.com
How to write a function that generates random string in Javascript?
To write a function that generates a random string in JavaScript, you can use the following code:
function generateRandomString(length) { let result = ''; const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; const charactersLength = characters.length; for (let i = 0; i < length; i++) { result += characters.charAt(Math.floor(Math.random() * charactersLength)); } return result; }
This function takes an argument length that specifies the length of the random string to generate. It generates the string by selecting random characters from a set of upper and lower case letters and numbers, and concatenating them together.
You can then use the function by calling it and passing in the desired length of the random string, like this:
const randomString = generateRandomString(10); // generates a random string of length 10
Did this tutorial help a little? How about buy me a cup of coffee?
Please feel free to use the comments form below if you have any questions or need more explanation on anything. I do not guarantee a response.
IMPORTANT: You must thoroughy test any instructions on a production-like test environment first before trying anything on production systems. And, make sure it is tested for security, privacy, and safety. See our terms here.
tags cloud
popular searches
free download for mysql database server 5.1.5, bison, gearman, source code, php, install cairo, java, mysql, install mysql, laptop, linux, mysql mysql, mysql initialization, tools, ubuntu
Similar Tutorials:
- Trim whitespaces from both ends of a string, using Javascript
- Sleep/Pause for specified number of seconds
- How to execute a function at regular intervals using Javascript
- Removing Whitespaces from Beginning of a String (Left Trim), using Javascript
- Which frontend framework should i learn?
Tutorials in 'Web Development > Javascript' (more):
- Which frontend framework should i learn?
- Bundle, validate, compress javascript files
- How to execute a function at regular intervals using Javascript
- Go to Top of the Page Using Javascript
- Sleep/Pause for specified number of seconds
Comments (write a comment):
0 comments so far. Be the first one to leave a comment on this article.