
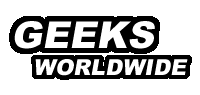
- Welcome to Geeksww.com
Simple Coding Style for PHP - Part 1/2
Many programmers use different kinds of coding styles to write code I have my own version that (i think) is simple and easy-to-use.
Code Formatting and Layout:
Code Formatting and Layout – which includes indentation, line length, use of white space, and use of Structured Query Language (SQL).Use Soft Tabs (soft tabs are not actually tabs, each soft tab is represented by a certain number of regular spaces) instead of Hard Tabs (regular tab). The reason is that tab spacing is different for different editors. Tab width of five spaces is good.
Try to break up lines after every 80 characters because 80 characters is the standard width of a Unix terminal and is a reasonable width for printing to hard copy in a readable font.
Some examples:
if ($month == 'september' || $month == 'april' || $month == 'june' || $month == 'november') { return 30; }
This should be changed to following:
if ($month == 'september' || $month == 'april' || $month == 'june' || $month == 'november') { return 30; }
This method works equally well for functions’ parameters:
mail([email protected], "subject", $body, "from: rn");
SQL Guidelines:
We’ll follow the following rules:
- Capitalize keywords
- Break lines on keywords
- Use table aliases to keep the code clean
Example:
Consider the following query:$query = "select firstname, lastname from employees, departments where employees.dept_id = department.dept_id and department.name = ‘Arts’";
Above should be changed to following:
$query = "SELECT firstname, lastname FROM employees e, departments d WHERE e.dept_id = d.dept_id AND d.name = ‘Arts’";
Using braces in control structures (e.g. if, for, foreach, while):
To avoid confusion, we’ll always use braces, even when only a single statement is being conditionally executed.
if( isset ($name)) echo "hello $name";
Must be changed to following:
if( isset ($name)) { echo "hello $name"; }
Consistency in using braces:
We’ll use BSD style braces for conditional statements. In BSD style braces the braces are placed on the line following the conditional with the braces outdented to align with the keyword. Follow the same rule for functions.
if ($conditional) { //statement }
Page: 1 2
Did this tutorial help a little? How about buy me a cup of coffee?
Please feel free to use the comments form below if you have any questions or need more explanation on anything. I do not guarantee a response.
IMPORTANT: You must thoroughy test any instructions on a production-like test environment first before trying anything on production systems. And, make sure it is tested for security, privacy, and safety. See our terms here.
tags cloud
popular searches
free download for mysql database server 5.1.5, bison, gearman, source code, php, install cairo, java, mysql, install mysql, laptop, linux, mysql mysql, mysql initialization, tools, ubuntu
Similar Tutorials:
- PHP Useful functions (Part 1) - The inRange function
- Simple Coding Style for PHP - Part 2/2
- Installing Gearman shared PECL extension for PHP on Debian/Ubuntu Linux
- Generating prime nos in PHP using multiprocessing - Geaman with PHP client Part I
- How to check PHP version number?
Tutorials in 'Web Development > PHP' (more):
- Generating prime nos in PHP using multiprocessing - Geaman with PHP client Part I
- PHP Useful functions (Part 2) - The currentURL function
- PHP Useful functions (Part 1) - The inRange function
- Generating Unique IDs in PHP
- How to check PHP version number?
Comments (write a comment):
0 comments so far. Be the first one to leave a comment on this article.