
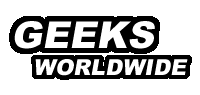
- Welcome to Geeksww.com
Bash script to compare remote directory's files using file size
Here is a bash script that compares two remote directories and their subdirectories using file size to check for differences:
#!/bin/bash # Set the hostnames and username for the two remote servers hostname1="remote_server_1" hostname2="remote_server_2" username="remote_user" # Set the paths to the directories on the two remote servers dir1="/path/to/remote/directory/1" dir2="/path/to/remote/directory/2" # Use ssh to execute the 'find' command on each remote server and store the output in a local file ssh $username@$hostname1 "find $dir1 -type f" > dir1_files.txt ssh $username@$hostname2 "find $dir2 -type f" > dir2_files.txt # Compare the two files line by line to check for differences while read line1 <&3 && read line2 <&4; do # Get the file sizes for the current files size1=$(ssh $username@$hostname1 "stat -c %s $line1") size2=$(ssh $username@$hostname2 "stat -c %s $line2") # Compare the file sizes if [ $size1 -ne $size2 ]; then # The file sizes are different, so the files are different echo "$line1 and $line2 are different" fi done 3<dir1_files.txt 4<dir2_files.txt # Clean up by deleting the temporary files rm dir1_files.txt rm dir2_files.txt
This script uses the find command to list all of the files in the dir1 and dir2 directories on the two remote servers, and stores the output in local files dir1_files.txt and dir2_files.txt, respectively. It then uses a while loop to read the two files line by line and compare the file sizes for each pair of files. If the file sizes are different, it prints a message indicating that the files are different. Finally, it cleans up by deleting the temporary files.
Note that this script does not compare the contents of the files, only their sizes. If you want to compare the contents of the files, you can use the cmp command in a similar way as in the previous example.
Did this tutorial help a little? How about buy me a cup of coffee?
Please feel free to use the comments form below if you have any questions or need more explanation on anything. I do not guarantee a response.
IMPORTANT: You must thoroughy test any instructions on a production-like test environment first before trying anything on production systems. And, make sure it is tested for security, privacy, and safety. See our terms here.
tags cloud
popular searches
free download for mysql database server 5.1.5, bison, gearman, source code, php, install cairo, java, mysql, install mysql, laptop, linux, mysql mysql, mysql initialization, tools, ubuntu
Similar Tutorials:
- Install MySQL Server 5.0 and 5.1 from source code
- How to setup Spamassasin to run as a deamon?
- Configuring Ubuntu Linux After Installation
- setfacl: command not found
- How to download, compile, and install GNU ncurses on Debian/Ubuntu Linux?
Tutorials in 'Operating Systems > Linux' (more):
- Script to transfer files to remote server with verification
- setfacl: command not found
- How to download, compile, and install CMake on Linux
- How to download, compile, and install GNU ncurses on Debian/Ubuntu Linux?
- How to install libevent on Debian/Ubuntu/Centos Linux?
Comments (write a comment):
0 comments so far. Be the first one to leave a comment on this article.