
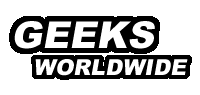
- Welcome to Geeksww.com
How to create a constructor that clones another object in Dart
Here is an example of how you can create a constructor that clones an object in Dart:
class MyClass { int _id; String _name; MyClass(this._id, this._name); // Clone constructor MyClass.clone(MyClass other) : this._id = other._id, this._name = other._name; } void main() { var original = MyClass(1, 'Original'); var clone = MyClass.clone(original); }
In this example, the MyClass
class has a private field _id
and a private field _name
. The main constructor takes two arguments, _id
and _name
, and initializes the corresponding fields.
The MyClass.clone()
constructor is a named constructor that takes another MyClass object as an argument and initializes the new object's fields with the values from the other object. This allows you to create a new object that is a copy of the original object.
To use the clone constructor, you can create an instance of MyClass using the main constructor, and then use the clone constructor to create a new instance of MyClass that is a copy of the original.
Did this tutorial help a little? How about buy me a cup of coffee?
Please feel free to use the comments form below if you have any questions or need more explanation on anything. I do not guarantee a response.
IMPORTANT: You must thoroughy test any instructions on a production-like test environment first before trying anything on production systems. And, make sure it is tested for security, privacy, and safety. See our terms here.
tags cloud
popular searches
free download for mysql database server 5.1.5, bison, gearman, source code, php, install cairo, java, mysql, install mysql, laptop, linux, mysql mysql, mysql initialization, tools, ubuntu
Similar Tutorials:
- How to create and maintain a fixed length queue in dart?
- Pass an object through navigator.pushnamed using provider
- Options to store data locally in a flutter app
- ?? - the null-coalescing operator in Dart
- Dart - x positional argument(s) expected, but 0 found.
Tutorials in 'Web Development > Dart' (more):
- How to create and maintain a fixed length queue in dart?
- Options to store data locally in a flutter app
- Dart - x positional argument(s) expected, but 0 found.
- ?? - the null-coalescing operator in Dart
- Pass an object through navigator.pushnamed using provider
Comments (write a comment):
0 comments so far. Be the first one to leave a comment on this article.